How to Execute HTTP POST Requests in Android
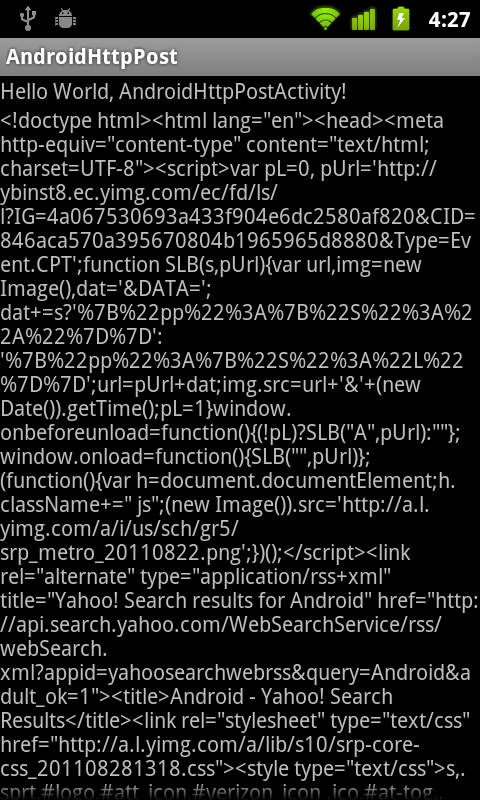
Internet connectivity is one of the main reasons behind the popularity of smart-phones. The ability to browse the web and access ones favourite content is what attracts most people towards these devices. Android being the most popular platform, allows the use of several network technologies for data services. However, browsing on a device is just the tip of the iceberg, and with over 600,000 apps on the Play Store, Android devices have much more to offer. Most of these apps make use of the device’s internet connectivity to bring added value. For instance, social networking apps like Twitter and Facebook need internet access to sync. Even the most basic weather widget needs data connection for updates.
However, in order for the app to utilize data, it needs to make HTTP requests. If you are looking to add this functionality to your app, you will need Java IDE, Android development tools (SDK etc), an Android device with internet connectivity for testing.
Instructions
-
1
Every Android app (Application package file format) needs to have an ‘AndroidManifest.xml’ file in the root directory, which declares all important information the system needs to have in order to execute the app’s code. The manifest is where you need to declare permission for internet using the following code:
-
2
Now you need to create the HTTPClient and Post objects in order to execute the request. The address object will be your POST’s destination (any URL).
// Creating HTTP client
HttpClient httpClient = new DefaultHttpClient();
// Creating HTTP Post
HttpPost httpPost = new HttpPost("http://www.abc.com/xyz"); -
3
Post parameters need to be set now. The Post needs to process data, and needs NameValuePairs.
// Building post parameters, key and value pair
List nameValuePair = new ArrayList(2);
nameValuePair.add(new BasicNameValuePair("email", "user@xyz.com"));
nameValuePair.add(new BasicNameValuePair("password", "encrypted_password"));
Before executing the POST request, the data needs to be encoded to convert strings into URL format.
// Url Encoding the POST parameters
try {
httpPost.setEntity(new UrlEncodedFormEntity(nameValuePair));
}
catch (UnsupportedEncodingException e) {
// writing error to Log
e.printStackTrace();
} -
4
Now you can execute the POST request with the HTTPClient created earlier.
// Making HTTP Request
try {
HttpResponse response = httpClient.execute(httpPost);
// writing response to log
Log.d("Http Response:", response.toString());
} catch (ClientProtocolException e) {
// writing exception to log
e.printStackTrace();
} catch (IOException e) {
// writing exception to log
e.printStackTrace();
}