How to Write a for Loop in Java
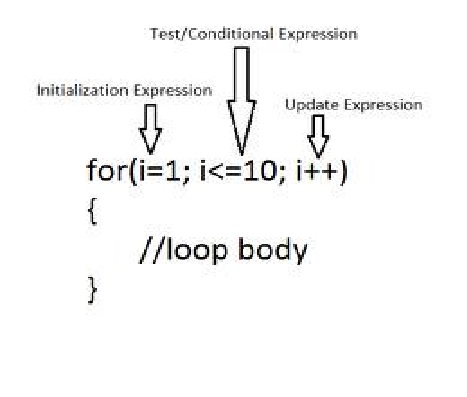
Loops in programming languages are control statements that are used to perform a task iteratively, but in exactly the specified number of times. Just like any other programming language, Java also offers this in the form of three looping mechanisms.
These looping mechanisms help us solve problems that would otherwise not be that straightforward. All of these looping mechanisms have their own benefits but the ‘for loop’ is simplest of all. As the name suggests the ‘for loop’ is a control structure that repeats a task until the specified number of times.
Here is the syntax:
for(initialization; Boolean_expression; increment)
{
//Statements
}
The control statements are written within the circle brackets and the statements to be looped through are written within the parenthesis after the control statements.
Instructions
-
1
Write the for loop in your Java program where you want iteration.
-
2
The next step is to write the control statements. Before that however, lets recall some basics. It takes three statements inside it; the first one is the initialization statement which shows where you want the loop to start from. The second one is the Boolean expression that will specify where you want your loop to end. The last one will tell how many times you want your statement to loop.
-
3
Now you will initialize the variable. To make things simpler this is explained with the help of an example. Suppose that we want to printout 1,3,5,7,9. So firstly we will declare a variable with the name counter which is of integer type and then we initialize it with 1. This will start our loop with number 1.
Next, according to the requirement, it will loop through 9 times and not more then that. So here we will have another statement which is counter<=9. The next thing is to specify how much we want to increment it by.
According to our requirement, it must be incremented by 2 at a time. So we will write it like counter +2. Now we are done with the three parameters that the 'for loop' will take.
Here's how it will be written:
for(int counter=1; counter<=10; counter+=2) -
4
Next is the statement we want to execute.
We will write it like this - System.out.printIn(counter).
This statement will printout the values one after the other according to the sequence mentioned as counter variable.
Now run this and we get a result that must be 1,3,5,7,9.
When it reaches 10, it checks the condition seeing that the loop has reached 10 and if the variable is incremented further by 2 it will volate the condition which is counter<=10.
So here it proves false and will stop executing. Upon this the control will come out of the loop and the final result will be displayed to the user.
-
5
for(int counter=1; counter<=10; counter+=2)
{
System.out.printIn(counter);
}
Result:
1
3
5
7
9